Fuzzy String Matching for Document Standardization
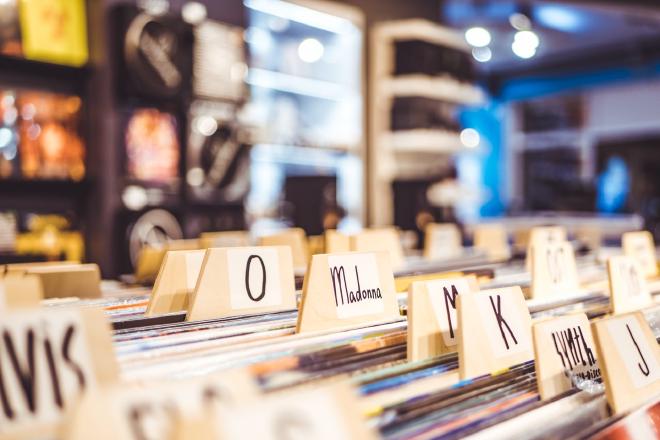
Sometimes a case occurs where you need to match text data to some expected text format but may not be able to because of misspellings or typos. This was the case for me in a work project from some time back, where I needed to match street addresses for different countries to a precise format for every country. There was also the problem of omissions in some address formats, such as the lack of United Kingdom or UK for London, England for example, or the problem of variations on USA, US, United States or United States of America. What came to my rescue was the approximate string matching, or fuzzy string searching technique.
At the time of the project I used a package called FuzzyWuzzy, which has been more recently rebranded as TheFuzz. This technique uses a string metric called the Levenshtein distance which calculates the differences in spelling between two text strings and determines how far apart in spelling the two strings are. There are many articles, such as this one, that gives a good breakdown of the topic of fuzzy matching in Python, and how it can be used to make data reach certain quality standards.
The problem for me at the time was that I needed to convert address listings into the correct format for long list of addresses in CSV files, some of which spanned several tens of thousands of lines. For this process unfortunately the FuzzyWuzzy package was painfully slow at finding the correct spelling. To be fair the package wasn’t the sole element responsible for the slowdown, a lot of optimization was also needed for pandas, which was the main tool I was using to extract, transform and glue the data together.
Various strategies and optimizations are required to improve the time of calculation for fuzzy string matching in text documents. Here I summarize a series of steps to consider when working on problems of this kind that can speed up the process:
-
Use Efficient Algorithms: Instead of using brute-force methods for string matching, employ more efficient algorithms like the Levenshtein distance, Jaccard similarity, or cosine similarity. These algorithms have lower time complexity and can significantly speed up the fuzzy matching process.
-
Indexing and Preprocessing: Create indexes or data structures (e.g., suffix arrays, tries, or n-grams) to speed up the search for potential matches. Preprocessing the text documents to generate these structures allows for faster lookup and reduces the number of comparisons needed.
-
Parallel Processing: Utilize multi-core CPUs or distributed computing to parallelize the fuzzy string matching process. Break the matching task into smaller chunks and process them simultaneously on different cores or machines, leading to faster overall computation.
-
Vectorization: Use vectorized operations and libraries like NumPy in Python to perform operations on multiple data points simultaneously. Vectorized calculations can be more efficient than iterating through each element in a loop.
-
Approximate Matching Libraries: Instead of implementing fuzzy string matching algorithms from scratch, consider using specialized libraries or tools that are highly optimized for performance. I have already mentioned TheFuzz, but there are also other packages in Python such as rapidfuzz.
-
Reduce Comparison Scope: Employ heuristics or filtering mechanisms to narrow down the number of potential matches before performing the full fuzzy string matching. For example, you can use string length or token-based filtering to exclude unlikely candidates.
-
Limiting Comparison Threshold: Set a threshold for similarity scores to exclude matches that fall below it. This can significantly reduce the number of comparisons needed, especially for approximate matching with low similarity requirements. Personally for what I had seen working on my project at the time, this helped a lot in improving fuzzy string matching performance.
-
Caching: Cache results of previously computed string matching operations, so you don’t need to recompute them if the same strings or similar strings appear again. This can save time in repeated fuzzy matching scenarios. This is a well-known optimization technique of computer science known as memoization.
-
Data Reduction: If applicable, reduce the size of text documents by removing irrelevant or redundant information before conducting the fuzzy matching. This reduces the input data size and can speed up the calculations. This wasn’t applicable in my case at the time, since I had a very specific data set to work with, but in general it can be a useful step forward.
-
Optimized Data Structures: Depending on the specific algorithm used for fuzzy string matching, ensure that you’re using the most suitable data structures for the task. For example, a compact and efficient data structure for representing strings might improve performance.
On a final note, deep learning can be employed to quicken calculations in some cases, but it’s important to note that it may not always be the most efficient or practical solution, especially for fuzzy string matching tasks. For certain algorithms and libraries, you might leverage graphics processing units (GPUs) to perform computations in parallel, which can result in substantial speed improvements.
By combining these techniques and choosing the right algorithms, libraries, and data structures, one can significantly improve the time of calculation for fuzzy string matching in text documents. The specific approach may vary depending on the nature of your data and the requirements of your application.
Happy fuzzy string matching!